Cohere Chat
提供 Bedrock Cohere 聊天模型。將生成式 AI 功能整合到關鍵應用程式和工作流程中,以改善業務成果。
AWS Bedrock Cohere 模型頁面 和 Amazon Bedrock 使用者指南 包含有關如何使用 AWS 託管模型的詳細資訊。
先決條件
請參閱 Spring AI 文件中有關 Amazon Bedrock 的章節,以了解如何設定 API 存取權限。
自動配置
將 spring-ai-bedrock-ai-spring-boot-starter
依賴項新增到您專案的 Maven pom.xml
檔案中
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock-ai-spring-boot-starter</artifactId>
</dependency>
或您的 Gradle build.gradle
建置檔案中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock-ai-spring-boot-starter'
}
請參閱依賴管理章節,將 Spring AI BOM 新增到您的建置檔案中。 |
啟用 Cohere Chat 支援
預設情況下,Cohere 模型是停用的。若要啟用它,請將 spring.ai.bedrock.cohere.chat.enabled
屬性設定為 true
。匯出環境變數是設定此配置屬性的一種方式
export SPRING_AI_BEDROCK_COHERE_CHAT_ENABLED=true
Chat 屬性
前綴 spring.ai.bedrock.aws
是用於配置與 AWS Bedrock 連線的屬性前綴。
屬性 | 描述 | 預設值 |
---|---|---|
spring.ai.bedrock.aws.region |
要使用的 AWS 區域。 |
us-east-1 |
spring.ai.bedrock.aws.timeout |
要使用的 AWS 超時時間。 |
5m |
spring.ai.bedrock.aws.access-key |
AWS 存取金鑰。 |
- |
spring.ai.bedrock.aws.secret-key |
AWS 密碼金鑰。 |
- |
前綴 spring.ai.bedrock.cohere.chat
是用於配置 Cohere 聊天模型實作的屬性前綴。
屬性 | 描述 | 預設值 |
---|---|---|
spring.ai.bedrock.cohere.chat.enabled |
啟用或停用 Cohere 支援 |
false |
spring.ai.bedrock.cohere.chat.model |
要使用的模型 ID。請參閱 CohereChatModel 以了解支援的模型。 |
cohere.command-text-v14 |
spring.ai.bedrock.cohere.chat.options.temperature |
控制輸出的隨機性。值範圍為 [0.0,1.0] |
0.7 |
spring.ai.bedrock.cohere.chat.options.topP |
在取樣時要考慮的權杖的最大累積機率。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.topK |
指定模型用於產生下一個權杖的權杖選項數量 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.maxTokens |
指定在產生的回應中使用的最大權杖數。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.stopSequences |
配置模型可辨識的最多四個序列。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.returnLikelihoods |
權杖可能性會與回應一起傳回。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.numGenerations |
模型應傳回的最大生成次數。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.logitBias |
防止模型產生不想要的權杖,或激勵模型包含想要的權杖。 |
AWS Bedrock 預設值 |
spring.ai.bedrock.cohere.chat.options.truncate |
指定 API 如何處理長度超過最大權杖長度的輸入 |
AWS Bedrock 預設值 |
請查看 CohereChatModel 以了解其他模型 ID。支援的值為:cohere.command-light-text-v14
和 cohere.command-text-v14
。模型 ID 值也可以在 AWS Bedrock 文件中的基礎模型 ID 中找到。
所有以 spring.ai.bedrock.cohere.chat.options 為前綴的屬性都可以在執行時透過將請求特定的 執行時選項 新增到 Prompt 呼叫中來覆寫。 |
執行時選項
BedrockCohereChatOptions.java 提供了模型配置,例如 temperature、topK、topP 等。
在啟動時,可以使用 BedrockCohereChatModel(api, options)
建構子或 spring.ai.bedrock.cohere.chat.options.*
屬性來配置預設選項。
在執行時,您可以透過將新的、請求特定的選項新增到 Prompt
呼叫中來覆寫預設選項。例如,若要覆寫特定請求的預設 temperature
ChatResponse response = chatModel.call(
new Prompt(
"Generate the names of 5 famous pirates.",
BedrockCohereChatOptions.builder()
.withTemperature(0.4)
.build()
));
除了模型特定的 BedrockCohereChatOptions 之外,您還可以使用可攜式的 ChatOptions 實例,使用 ChatOptionsBuilder#builder() 建立。 |
範例控制器
建立 新的 Spring Boot 專案,並將 spring-ai-bedrock-ai-spring-boot-starter
新增到您的 pom(或 gradle)依賴項中。
在 src/main/resources
目錄下新增 application.properties
檔案,以啟用和配置 Cohere 聊天模型
spring.ai.bedrock.aws.region=eu-central-1
spring.ai.bedrock.aws.timeout=1000ms
spring.ai.bedrock.aws.access-key=${AWS_ACCESS_KEY_ID}
spring.ai.bedrock.aws.secret-key=${AWS_SECRET_ACCESS_KEY}
spring.ai.bedrock.cohere.chat.enabled=true
spring.ai.bedrock.cohere.chat.options.temperature=0.8
將 regions 、access-key 和 secret-key 替換為您的 AWS 憑證。 |
這將建立一個 BedrockCohereChatModel
實作,您可以將其注入到您的類別中。以下是一個簡單的 @Controller
類別範例,該類別使用聊天模型進行文字生成。
@RestController
public class ChatController {
private final BedrockCohereChatModel chatModel;
@Autowired
public ChatController(BedrockCohereChatModel chatModel) {
this.chatModel = chatModel;
}
@GetMapping("/ai/generate")
public Map generate(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
return Map.of("generation", this.chatModel.call(message));
}
@GetMapping("/ai/generateStream")
public Flux<ChatResponse> generateStream(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
Prompt prompt = new Prompt(new UserMessage(message));
return this.chatModel.stream(prompt);
}
}
手動配置
BedrockCohereChatModel 實作了 ChatModel
和 StreamingChatModel
,並使用 Low-level CohereChatBedrockApi Client 連接到 Bedrock Cohere 服務。
將 spring-ai-bedrock
依賴項新增到您專案的 Maven pom.xml
檔案中
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock</artifactId>
</dependency>
或您的 Gradle build.gradle
建置檔案中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock'
}
請參閱依賴管理章節,將 Spring AI BOM 新增到您的建置檔案中。 |
接下來,建立一個 BedrockCohereChatModel 並使用它進行文字生成
CohereChatBedrockApi api = new CohereChatBedrockApi(CohereChatModel.COHERE_COMMAND_V14.id(),
EnvironmentVariableCredentialsProvider.create(),
Region.US_EAST_1.id(),
new ObjectMapper(),
Duration.ofMillis(1000L));
BedrockCohereChatModel chatModel = new BedrockCohereChatModel(this.api,
BedrockCohereChatOptions.builder()
.withTemperature(0.6)
.withTopK(10)
.withTopP(0.5)
.withMaxTokens(678)
.build());
ChatResponse response = this.chatModel.call(
new Prompt("Generate the names of 5 famous pirates."));
// Or with streaming responses
Flux<ChatResponse> response = this.chatModel.stream(
new Prompt("Generate the names of 5 famous pirates."));
Low-level CohereChatBedrockApi Client
CohereChatBedrockApi 提供了一個輕量級 Java 客户端,基於 AWS Bedrock Cohere Command 模型。
以下類別圖說明了 CohereChatBedrockApi 介面和建構區塊
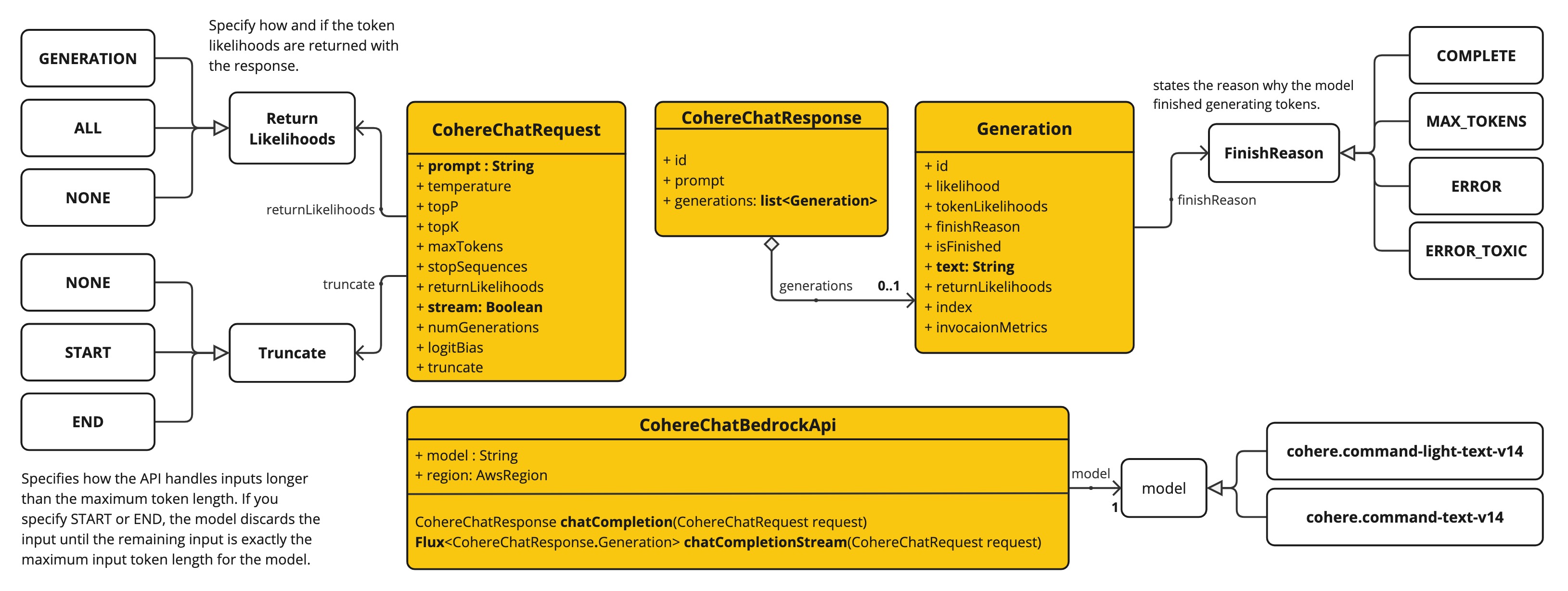
CohereChatBedrockApi 支援 cohere.command-light-text-v14
和 cohere.command-text-v14
模型,用於同步(例如 chatCompletion()
)和串流(例如 chatCompletionStream()
)請求。
以下是一個簡單的程式碼片段,說明如何以程式設計方式使用 API
CohereChatBedrockApi cohereChatApi = new CohereChatBedrockApi(
CohereChatModel.COHERE_COMMAND_V14.id(),
Region.US_EAST_1.id(),
Duration.ofMillis(1000L));
var request = CohereChatRequest
.builder("What is the capital of Bulgaria and what is the size? What is the national anthem?")
.withStream(false)
.withTemperature(0.5)
.withTopP(0.8)
.withTopK(15)
.withMaxTokens(100)
.withStopSequences(List.of("END"))
.withReturnLikelihoods(CohereChatRequest.ReturnLikelihoods.ALL)
.withNumGenerations(3)
.withLogitBias(null)
.withTruncate(Truncate.NONE)
.build();
CohereChatResponse response = this.cohereChatApi.chatCompletion(this.request);
var request = CohereChatRequest
.builder("What is the capital of Bulgaria and what is the size? What it the national anthem?")
.withStream(true)
.withTemperature(0.5)
.withTopP(0.8)
.withTopK(15)
.withMaxTokens(100)
.withStopSequences(List.of("END"))
.withReturnLikelihoods(CohereChatRequest.ReturnLikelihoods.ALL)
.withNumGenerations(3)
.withLogitBias(null)
.withTruncate(Truncate.NONE)
.build();
Flux<CohereChatResponse.Generation> responseStream = this.cohereChatApi.chatCompletionStream(this.request);
List<CohereChatResponse.Generation> responses = this.responseStream.collectList().block();