OAuth 2.0 資源伺服器不透明令牌
內省的最低相依性
如 JWT 的最低相依性 中所述,大多數資源伺服器支援都收集在 spring-security-oauth2-resource-server
中。但是,除非提供自訂的 OpaqueTokenIntrospector
,否則資源伺服器將會回退到 NimbusOpaqueTokenIntrospector。這表示為了擁有支援不透明 Bearer 令牌的最低限度可運作的資源伺服器,spring-security-oauth2-resource-server
和 oauth2-oidc-sdk
都是必要的。請參考 spring-security-oauth2-resource-server
以判斷 oauth2-oidc-sdk
的正確版本。
內省的最低設定
通常,不透明令牌可以透過授權伺服器託管的 OAuth 2.0 內省端點 進行驗證。當撤銷是一項需求時,這會非常方便。
當使用 Spring Boot 時,將應用程式設定為使用內省的資源伺服器包含兩個基本步驟。首先,包含所需的相依性,其次,指示內省端點詳細資訊。
指定授權伺服器
若要指定內省端點的位置,只需執行
spring:
security:
oauth2:
resourceserver:
opaque-token:
introspection-uri: https://idp.example.com/introspect
client-id: client
client-secret: secret
其中 idp.example.com/introspect
是由您的授權伺服器託管的內省端點,而 client-id
和 client-secret
是點擊該端點所需的憑證。
資源伺服器將使用這些屬性來進一步自我設定,並隨後驗證傳入的 JWT。
當使用內省時,授權伺服器的說法就是法律。如果授權伺服器回應令牌有效,那麼它就是有效的。 |
就這樣!
執行階段預期
應用程式啟動後,資源伺服器將嘗試處理任何包含 Authorization: Bearer
標頭的請求
GET / HTTP/1.1
Authorization: Bearer some-token-value # Resource Server will process this
只要指示此方案,資源伺服器就會嘗試根據 Bearer 令牌規範處理請求。
給定一個不透明令牌,資源伺服器將會
-
使用提供的憑證和令牌查詢提供的內省端點
-
檢查回應中是否有
{ 'active' : true }
屬性 -
將每個範圍對應到具有前綴
SCOPE_
的授權
預設情況下,產生的 Authentication#getPrincipal
是一個 Spring Security OAuth2AuthenticatedPrincipal
物件,而 Authentication#getName
則對應到令牌的 sub
屬性(如果存在)。
從這裡,您可能想要跳到
不透明令牌驗證如何運作
接下來,讓我們看看 Spring Security 用於支援基於 servlet 的應用程式(例如我們剛才看到的應用程式)中 不透明令牌 驗證的架構組件。
OpaqueTokenAuthenticationProvider
是一個 AuthenticationProvider
實作,它利用 OpaqueTokenIntrospector
來驗證不透明令牌。
讓我們看看 OpaqueTokenAuthenticationProvider
在 Spring Security 中是如何運作的。圖示說明了 讀取 Bearer 令牌 中的圖示中 AuthenticationManager
的運作細節。
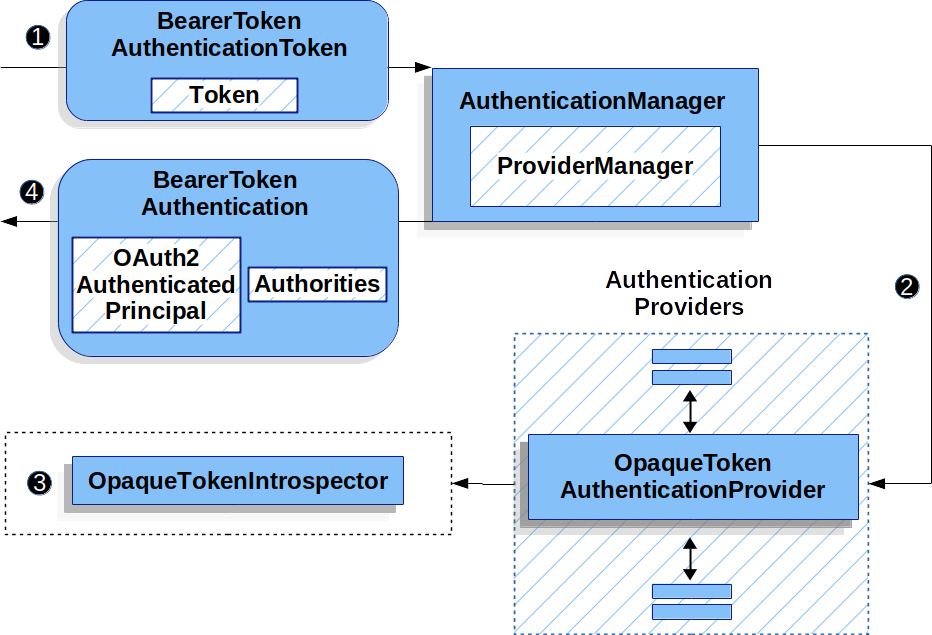
OpaqueTokenAuthenticationProvider
用法 來自 讀取 Bearer 令牌 的驗證
Filter
將 BearerTokenAuthenticationToken
傳遞給由 ProviderManager
實作的 AuthenticationManager
。
ProviderManager
設定為使用類型為 OpaqueTokenAuthenticationProvider
的 AuthenticationProvider。
OpaqueTokenAuthenticationProvider
內省不透明令牌,並使用 OpaqueTokenIntrospector
新增授與的授權。當驗證成功時,傳回的 Authentication
類型為 BearerTokenAuthentication
,且其主體是由設定的 OpaqueTokenIntrospector
傳回的 OAuth2AuthenticatedPrincipal
。最終,驗證 Filter
會將傳回的 BearerTokenAuthentication
設定在 SecurityContextHolder
上。
驗證後查找屬性
令牌驗證後,BearerTokenAuthentication
的實例會設定在 SecurityContext
中。
這表示當您在設定中使用 @EnableWebMvc
時,它可以在 @Controller
方法中使用
-
Java
-
Kotlin
@GetMapping("/foo")
public String foo(BearerTokenAuthentication authentication) {
return authentication.getTokenAttributes().get("sub") + " is the subject";
}
@GetMapping("/foo")
fun foo(authentication: BearerTokenAuthentication): String {
return authentication.tokenAttributes["sub"].toString() + " is the subject"
}
由於 BearerTokenAuthentication
持有 OAuth2AuthenticatedPrincipal
,這也表示它也可以在控制器方法中使用
-
Java
-
Kotlin
@GetMapping("/foo")
public String foo(@AuthenticationPrincipal OAuth2AuthenticatedPrincipal principal) {
return principal.getAttribute("sub") + " is the subject";
}
@GetMapping("/foo")
fun foo(@AuthenticationPrincipal principal: OAuth2AuthenticatedPrincipal): String {
return principal.getAttribute<Any>("sub").toString() + " is the subject"
}
透過 SpEL 查找屬性
當然,這也表示可以透過 SpEL 存取屬性。
例如,如果使用 @EnableGlobalMethodSecurity
以便可以使用 @PreAuthorize
註解,您可以執行
-
Java
-
Kotlin
@PreAuthorize("principal?.attributes['sub'] == 'foo'")
public String forFoosEyesOnly() {
return "foo";
}
@PreAuthorize("principal?.attributes['sub'] == 'foo'")
fun forFoosEyesOnly(): String {
return "foo"
}
覆寫或取代 Boot 自動設定
Spring Boot 代表資源伺服器產生兩個 @Bean
。
第一個是將應用程式設定為資源伺服器的 SecurityFilterChain
。當使用不透明令牌時,此 SecurityFilterChain
看起來像
-
Java
-
Kotlin
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(OAuth2ResourceServerConfigurer::opaqueToken);
return http.build();
}
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
opaqueToken { }
}
}
return http.build()
}
如果應用程式未公開 SecurityFilterChain
bean,則 Spring Boot 將會公開上述預設 bean。
取代它就像在應用程式中公開 bean 一樣簡單
-
Java
-
Kotlin
import static org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
public class MyCustomSecurityConfiguration {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.requestMatchers("/messages/**").access(hasScope("message:read"))
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.opaqueToken(opaqueToken -> opaqueToken
.introspector(myIntrospector())
)
);
return http.build();
}
}
import org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
class MyCustomSecurityConfiguration {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize("/messages/**", hasScope("SCOPE_message:read"))
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
opaqueToken {
introspector = myIntrospector()
}
}
}
return http.build()
}
}
上述程式碼需要 message:read
範圍才能用於任何以 /messages/
開頭的 URL。
oauth2ResourceServer
DSL 上的方法也會覆寫或取代自動設定。
例如,Spring Boot 建立的第二個 @Bean
是 OpaqueTokenIntrospector
,它將 String
令牌解碼為 OAuth2AuthenticatedPrincipal
的已驗證實例
-
Java
-
Kotlin
@Bean
public OpaqueTokenIntrospector introspector() {
return new NimbusOpaqueTokenIntrospector(introspectionUri, clientId, clientSecret);
}
@Bean
fun introspector(): OpaqueTokenIntrospector {
return NimbusOpaqueTokenIntrospector(introspectionUri, clientId, clientSecret)
}
如果應用程式未公開 OpaqueTokenIntrospector
bean,則 Spring Boot 將會公開上述預設 bean。
而且可以使用 introspectionUri()
和 introspectionClientCredentials()
覆寫其設定,或使用 introspector()
取代其設定。
如果應用程式未公開 OpaqueTokenAuthenticationConverter
bean,則 spring-security 將會建置 BearerTokenAuthentication
。
或者,如果您完全未使用 Spring Boot,則所有這些組件(篩選器鏈、OpaqueTokenIntrospector
和 OpaqueTokenAuthenticationConverter
)都可以在 XML 中指定。
篩選器鏈的指定方式如下
-
Xml
<http>
<intercept-uri pattern="/**" access="authenticated"/>
<oauth2-resource-server>
<opaque-token introspector-ref="opaqueTokenIntrospector"
authentication-converter-ref="opaqueTokenAuthenticationConverter"/>
</oauth2-resource-server>
</http>
而 OpaqueTokenIntrospector
的指定方式如下
-
Xml
<bean id="opaqueTokenIntrospector"
class="org.springframework.security.oauth2.server.resource.introspection.NimbusOpaqueTokenIntrospector">
<constructor-arg value="${spring.security.oauth2.resourceserver.opaquetoken.introspection_uri}"/>
<constructor-arg value="${spring.security.oauth2.resourceserver.opaquetoken.client_id}"/>
<constructor-arg value="${spring.security.oauth2.resourceserver.opaquetoken.client_secret}"/>
</bean>
而 OpaqueTokenAuthenticationConverter
的指定方式如下
-
Xml
<bean id="opaqueTokenAuthenticationConverter"
class="com.example.CustomOpaqueTokenAuthenticationConverter"/>
使用 introspectionUri()
授權伺服器的內省 URI 可以 作為組態屬性 設定,也可以在 DSL 中提供
-
Java
-
Kotlin
-
Xml
@Configuration
@EnableWebSecurity
public class DirectlyConfiguredIntrospectionUri {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.opaqueToken(opaqueToken -> opaqueToken
.introspectionUri("https://idp.example.com/introspect")
.introspectionClientCredentials("client", "secret")
)
);
return http.build();
}
}
@Configuration
@EnableWebSecurity
class DirectlyConfiguredIntrospectionUri {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
opaqueToken {
introspectionUri = "https://idp.example.com/introspect"
introspectionClientCredentials("client", "secret")
}
}
}
return http.build()
}
}
<bean id="opaqueTokenIntrospector"
class="org.springframework.security.oauth2.server.resource.introspection.NimbusOpaqueTokenIntrospector">
<constructor-arg value="https://idp.example.com/introspect"/>
<constructor-arg value="client"/>
<constructor-arg value="secret"/>
</bean>
使用 introspectionUri()
的優先順序高於任何組態屬性。
使用 introspector()
比 introspectionUri()
更強大的是 introspector()
,它將完全取代 OpaqueTokenIntrospector
的任何 Boot 自動設定
-
Java
-
Kotlin
-
Xml
@Configuration
@EnableWebSecurity
public class DirectlyConfiguredIntrospector {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.opaqueToken(opaqueToken -> opaqueToken
.introspector(myCustomIntrospector())
)
);
return http.build();
}
}
@Configuration
@EnableWebSecurity
class DirectlyConfiguredIntrospector {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
opaqueToken {
introspector = myCustomIntrospector()
}
}
}
return http.build()
}
}
<http>
<intercept-uri pattern="/**" access="authenticated"/>
<oauth2-resource-server>
<opaque-token introspector-ref="myCustomIntrospector"/>
</oauth2-resource-server>
</http>
公開 OpaqueTokenIntrospector
@Bean
或者,公開 OpaqueTokenIntrospector
@Bean
具有與 introspector()
相同的效果
@Bean
public OpaqueTokenIntrospector introspector() {
return new NimbusOpaqueTokenIntrospector(introspectionUri, clientId, clientSecret);
}
設定授權
OAuth 2.0 內省端點通常會傳回 scope
屬性,指示已授與的範圍(或授權),例如
{ …, "scope" : "messages contacts"}
在這種情況下,資源伺服器將嘗試將這些範圍強制轉換為授與的授權清單,並在每個範圍前面加上字串 "SCOPE_"。
這表示若要使用從不透明令牌衍生的範圍來保護端點或方法,則對應的運算式應包含此前綴
-
Java
-
Kotlin
-
Xml
import static org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
public class MappedAuthorities {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorizeRequests -> authorizeRequests
.requestMatchers("/contacts/**").access(hasScope("contacts"))
.requestMatchers("/messages/**").access(hasScope("messages"))
.anyRequest().authenticated()
)
.oauth2ResourceServer(OAuth2ResourceServerConfigurer::opaqueToken);
return http.build();
}
}
import org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope
@Configuration
@EnableWebSecurity
class MappedAuthorities {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize("/contacts/**", hasScope("contacts"))
authorize("/messages/**", hasScope("messages"))
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
opaqueToken { }
}
}
return http.build()
}
}
<http>
<intercept-uri pattern="/contacts/**" access="hasAuthority('SCOPE_contacts')"/>
<intercept-uri pattern="/messages/**" access="hasAuthority('SCOPE_messages')"/>
<oauth2-resource-server>
<opaque-token introspector-ref="opaqueTokenIntrospector"/>
</oauth2-resource-server>
</http>
或類似的方法安全
-
Java
-
Kotlin
@PreAuthorize("hasAuthority('SCOPE_messages')")
public List<Message> getMessages(...) {}
@PreAuthorize("hasAuthority('SCOPE_messages')")
fun getMessages(): List<Message?> {}
手動擷取授權
預設情況下,不透明令牌支援將從內省回應中擷取 scope 宣告,並將其剖析為個別的 GrantedAuthority
實例。
例如,如果內省回應是
{
"active" : true,
"scope" : "message:read message:write"
}
然後資源伺服器將產生一個具有兩個授權的 Authentication
,一個用於 message:read
,另一個用於 message:write
。
當然,這可以使用自訂的 OpaqueTokenIntrospector
進行自訂,該自訂的內省器會查看屬性集並以自己的方式進行轉換
-
Java
-
Kotlin
public class CustomAuthoritiesOpaqueTokenIntrospector implements OpaqueTokenIntrospector {
private OpaqueTokenIntrospector delegate =
new NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret");
public OAuth2AuthenticatedPrincipal introspect(String token) {
OAuth2AuthenticatedPrincipal principal = this.delegate.introspect(token);
return new DefaultOAuth2AuthenticatedPrincipal(
principal.getName(), principal.getAttributes(), extractAuthorities(principal));
}
private Collection<GrantedAuthority> extractAuthorities(OAuth2AuthenticatedPrincipal principal) {
List<String> scopes = principal.getAttribute(OAuth2IntrospectionClaimNames.SCOPE);
return scopes.stream()
.map(SimpleGrantedAuthority::new)
.collect(Collectors.toList());
}
}
class CustomAuthoritiesOpaqueTokenIntrospector : OpaqueTokenIntrospector {
private val delegate: OpaqueTokenIntrospector = NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret")
override fun introspect(token: String): OAuth2AuthenticatedPrincipal {
val principal: OAuth2AuthenticatedPrincipal = delegate.introspect(token)
return DefaultOAuth2AuthenticatedPrincipal(
principal.name, principal.attributes, extractAuthorities(principal))
}
private fun extractAuthorities(principal: OAuth2AuthenticatedPrincipal): Collection<GrantedAuthority> {
val scopes: List<String> = principal.getAttribute(OAuth2IntrospectionClaimNames.SCOPE)
return scopes
.map { SimpleGrantedAuthority(it) }
}
}
此後,只需將此自訂內省器公開為 @Bean
即可輕鬆設定
-
Java
-
Kotlin
@Bean
public OpaqueTokenIntrospector introspector() {
return new CustomAuthoritiesOpaqueTokenIntrospector();
}
@Bean
fun introspector(): OpaqueTokenIntrospector {
return CustomAuthoritiesOpaqueTokenIntrospector()
}
設定逾時
預設情況下,資源伺服器使用 30 秒的連線和 Socket 逾時,以便與授權伺服器協調。
在某些情況下,這可能太短。此外,它沒有考慮到更複雜的模式,例如退避和探索。
若要調整資源伺服器連線到授權伺服器的方式,NimbusOpaqueTokenIntrospector
接受 RestOperations
的實例
-
Java
-
Kotlin
@Bean
public OpaqueTokenIntrospector introspector(RestTemplateBuilder builder, OAuth2ResourceServerProperties properties) {
RestOperations rest = builder
.basicAuthentication(properties.getOpaquetoken().getClientId(), properties.getOpaquetoken().getClientSecret())
.setConnectTimeout(Duration.ofSeconds(60))
.setReadTimeout(Duration.ofSeconds(60))
.build();
return new NimbusOpaqueTokenIntrospector(introspectionUri, rest);
}
@Bean
fun introspector(builder: RestTemplateBuilder, properties: OAuth2ResourceServerProperties): OpaqueTokenIntrospector? {
val rest: RestOperations = builder
.basicAuthentication(properties.opaquetoken.clientId, properties.opaquetoken.clientSecret)
.setConnectTimeout(Duration.ofSeconds(60))
.setReadTimeout(Duration.ofSeconds(60))
.build()
return NimbusOpaqueTokenIntrospector(introspectionUri, rest)
}
將內省與 JWT 搭配使用
一個常見的問題是內省是否與 JWT 相容。Spring Security 的不透明令牌支援設計為不關心令牌的格式 — 它很樂意將任何令牌傳遞給提供的內省端點。
因此,假設您的需求要求您在每次請求時都與授權伺服器檢查,以防 JWT 已被撤銷。
即使您使用 JWT 格式作為令牌,您的驗證方法也是內省,這表示您會想要執行
spring:
security:
oauth2:
resourceserver:
opaque-token:
introspection-uri: https://idp.example.org/introspection
client-id: client
client-secret: secret
在這種情況下,產生的 Authentication
將會是 BearerTokenAuthentication
。對應的 OAuth2AuthenticatedPrincipal
中的任何屬性都將是內省端點傳回的任何內容。
但是,假設很奇怪的是,內省端點僅傳回令牌是否處於活動狀態。現在怎麼辦?
在這種情況下,您可以建立自訂的 OpaqueTokenIntrospector
,它仍然會點擊端點,但接著會更新傳回的主體,使其具有 JWT 宣告作為屬性
-
Java
-
Kotlin
public class JwtOpaqueTokenIntrospector implements OpaqueTokenIntrospector {
private OpaqueTokenIntrospector delegate =
new NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret");
private JwtDecoder jwtDecoder = new NimbusJwtDecoder(new ParseOnlyJWTProcessor());
public OAuth2AuthenticatedPrincipal introspect(String token) {
OAuth2AuthenticatedPrincipal principal = this.delegate.introspect(token);
try {
Jwt jwt = this.jwtDecoder.decode(token);
return new DefaultOAuth2AuthenticatedPrincipal(jwt.getClaims(), NO_AUTHORITIES);
} catch (JwtException ex) {
throw new OAuth2IntrospectionException(ex);
}
}
private static class ParseOnlyJWTProcessor extends DefaultJWTProcessor<SecurityContext> {
JWTClaimsSet process(SignedJWT jwt, SecurityContext context)
throws JOSEException {
return jwt.getJWTClaimsSet();
}
}
}
class JwtOpaqueTokenIntrospector : OpaqueTokenIntrospector {
private val delegate: OpaqueTokenIntrospector = NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret")
private val jwtDecoder: JwtDecoder = NimbusJwtDecoder(ParseOnlyJWTProcessor())
override fun introspect(token: String): OAuth2AuthenticatedPrincipal {
val principal = delegate.introspect(token)
return try {
val jwt: Jwt = jwtDecoder.decode(token)
DefaultOAuth2AuthenticatedPrincipal(jwt.claims, NO_AUTHORITIES)
} catch (ex: JwtException) {
throw OAuth2IntrospectionException(ex.message)
}
}
private class ParseOnlyJWTProcessor : DefaultJWTProcessor<SecurityContext>() {
override fun process(jwt: SignedJWT, context: SecurityContext): JWTClaimsSet {
return jwt.jwtClaimsSet
}
}
}
此後,只需將此自訂內省器公開為 @Bean
即可輕鬆設定
-
Java
-
Kotlin
@Bean
public OpaqueTokenIntrospector introspector() {
return new JwtOpaqueTokenIntrospector();
}
@Bean
fun introspector(): OpaqueTokenIntrospector {
return JwtOpaqueTokenIntrospector()
}
呼叫 /userinfo
端點
一般來說,資源伺服器不關心底層使用者,而是關心已授與的授權。
也就是說,有時將授權陳述與使用者綁定可能很有價值。
如果應用程式也使用 spring-security-oauth2-client
,並且已設定適當的 ClientRegistrationRepository
,那麼使用自訂的 OpaqueTokenIntrospector
就非常簡單。以下實作執行三件事
-
委派給內省端點,以確認令牌的有效性
-
查找與
/userinfo
端點關聯的適當用戶端註冊 -
叫用並傳回來自
/userinfo
端點的回應
-
Java
-
Kotlin
public class UserInfoOpaqueTokenIntrospector implements OpaqueTokenIntrospector {
private final OpaqueTokenIntrospector delegate =
new NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret");
private final OAuth2UserService oauth2UserService = new DefaultOAuth2UserService();
private final ClientRegistrationRepository repository;
// ... constructor
@Override
public OAuth2AuthenticatedPrincipal introspect(String token) {
OAuth2AuthenticatedPrincipal authorized = this.delegate.introspect(token);
Instant issuedAt = authorized.getAttribute(ISSUED_AT);
Instant expiresAt = authorized.getAttribute(EXPIRES_AT);
ClientRegistration clientRegistration = this.repository.findByRegistrationId("registration-id");
OAuth2AccessToken token = new OAuth2AccessToken(BEARER, token, issuedAt, expiresAt);
OAuth2UserRequest oauth2UserRequest = new OAuth2UserRequest(clientRegistration, token);
return this.oauth2UserService.loadUser(oauth2UserRequest);
}
}
class UserInfoOpaqueTokenIntrospector : OpaqueTokenIntrospector {
private val delegate: OpaqueTokenIntrospector = NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret")
private val oauth2UserService = DefaultOAuth2UserService()
private val repository: ClientRegistrationRepository? = null
// ... constructor
override fun introspect(token: String): OAuth2AuthenticatedPrincipal {
val authorized = delegate.introspect(token)
val issuedAt: Instant? = authorized.getAttribute(ISSUED_AT)
val expiresAt: Instant? = authorized.getAttribute(EXPIRES_AT)
val clientRegistration: ClientRegistration = repository!!.findByRegistrationId("registration-id")
val accessToken = OAuth2AccessToken(BEARER, token, issuedAt, expiresAt)
val oauth2UserRequest = OAuth2UserRequest(clientRegistration, accessToken)
return oauth2UserService.loadUser(oauth2UserRequest)
}
}
如果您未使用 spring-security-oauth2-client
,它仍然非常簡單。您只需要使用您自己的 WebClient
實例來叫用 /userinfo
-
Java
-
Kotlin
public class UserInfoOpaqueTokenIntrospector implements OpaqueTokenIntrospector {
private final OpaqueTokenIntrospector delegate =
new NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret");
private final WebClient rest = WebClient.create();
@Override
public OAuth2AuthenticatedPrincipal introspect(String token) {
OAuth2AuthenticatedPrincipal authorized = this.delegate.introspect(token);
return makeUserInfoRequest(authorized);
}
}
class UserInfoOpaqueTokenIntrospector : OpaqueTokenIntrospector {
private val delegate: OpaqueTokenIntrospector = NimbusOpaqueTokenIntrospector("https://idp.example.org/introspect", "client", "secret")
private val rest: WebClient = WebClient.create()
override fun introspect(token: String): OAuth2AuthenticatedPrincipal {
val authorized = delegate.introspect(token)
return makeUserInfoRequest(authorized)
}
}
無論哪種方式,在建立您的 OpaqueTokenIntrospector
之後,您應該將其發佈為 @Bean
以覆寫預設值
-
Java
-
Kotlin
@Bean
OpaqueTokenIntrospector introspector() {
return new UserInfoOpaqueTokenIntrospector(...);
}
@Bean
fun introspector(): OpaqueTokenIntrospector {
return UserInfoOpaqueTokenIntrospector(...)
}